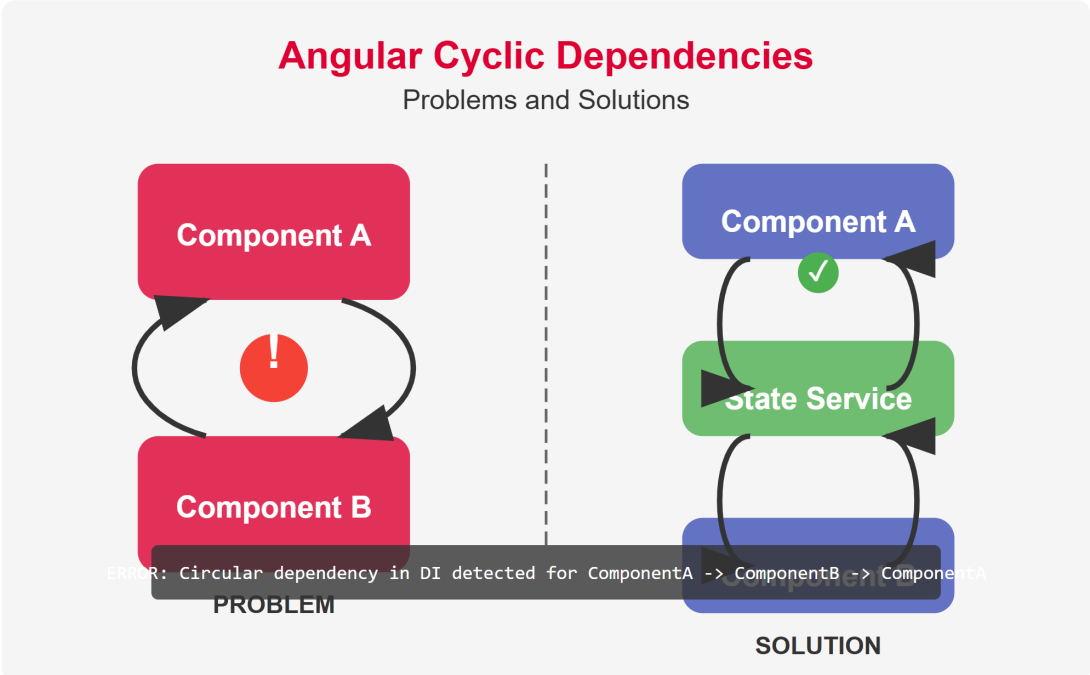
Introduction
In Angular applications, a cyclic dependency occurs when two or more components, services, or modules directly or indirectly depend on each other, creating a circular reference pattern. For example, if Component A depends on Service B, and Service B depends on Component A, you've created a cyclic dependency.
These circular references can cause significant problems:
- Runtime errors that are difficult to debug
- Memory leaks due to improper instantiation
- Unpredictable behavior in your application
- Initialization order problems
Cyclic dependencies often emerge organically as applications grow in complexity. They're particularly common in scenarios where components need to share functionality or communicate with each other, such as in feature-rich dashboards, complex forms with interdependent validation, or when implementing bidirectional data flows.
Identifying Cyclic Dependencies
Error Messages
When Angular encounters a cyclic dependency, it typically produces one of the following error messages:
- StaticInjectorError:
ERROR Error: Uncaught (in promise): NullInjectorError: R3InjectorError(AppModule)[ComponentA -> ServiceB -> ComponentA]: NullInjectorError: No provider for ComponentA!
- Circular dependency error:
ERROR Error: Circular dependency in DI detected for ComponentA -> ServiceB -> ComponentA
- Maximum call stack size exceeded:
RangeError: Maximum call stack size exceeded
Detection Tools
To proactively identify cyclic dependencies in your Angular projects:
- Angular Language Service: This VSCode or WebStorm extension can highlight potential dependency issues.
- Dependency Cruiser: A tool that creates visualizations of your dependency graph.
- Webpack Circular Dependency Plugin: Can be integrated into your build process to detect circular dependencies.
Common Causes of Cyclic Dependencies
Parent-Child Components
When parent and child components need to reference each other:
// parent.component.ts
import { ChildComponent } from './child.component';
@Component({...})
export class ParentComponent {
@ViewChild(ChildComponent) childComponent: ChildComponent;
}
// child.component.ts
import { ParentComponent } from './parent.component';
@Component({...})
export class ChildComponent {
constructor(private parent: ParentComponent) {}
}
Services Importing Each Other
// user.service.ts
import { AuthService } from './auth.service';
@Injectable()
export class UserService {
constructor(private authService: AuthService) {}
}
// auth.service.ts
import { UserService } from './user.service';
@Injectable()
export class AuthService {
constructor(private userService: UserService) {}
}
Modules with Circular Imports
// feature-a.module.ts
import { FeatureBModule } from './feature-b.module';
@NgModule({
imports: [FeatureBModule]
})
export class FeatureAModule {}
// feature-b.module.ts
import { FeatureAModule } from './feature-a.module';
@NgModule({
imports: [FeatureAModule]
})
export class FeatureBModule {}
Solutions and Best Practices
1. Interface Segregation
Create an interface to break the dependency cycle. This follows the Interface Segregation Principle from SOLID.
// shared-interfaces.ts
export interface UserInfo {
name: string;
isAuthenticated: boolean;
}
// user.service.ts
import { AuthService } from './auth.service';
import { UserInfo } from './shared-interfaces';
@Injectable()
export class UserService {
constructor(private authService: AuthService) {}
getUserInfo(): UserInfo {
return {
name: 'John Doe',
isAuthenticated: this.authService.isAuthenticated()
};
}
}
// auth.service.ts
import { UserInfo } from './shared-interfaces';
@Injectable()
export class AuthService {
private user: UserInfo | null = null;
setUser(user: UserInfo): void {
this.user = user;
}
isAuthenticated(): boolean {
return this.user ? this.user.isAuthenticated : false;
}
}
2. Using forwardRef()
Angular provides forwardRef()
to reference classes that haven't been defined yet. This is particularly useful for breaking cyclic dependencies in services.
// user.service.ts
import { Injectable, forwardRef, Inject } from '@angular/core';
import { AuthService } from './auth.service';
@Injectable()
export class UserService {
constructor(@Inject(forwardRef(() => AuthService)) private authService: AuthService) {}
getUserDetails() {
return { name: 'John', isAuthenticated: this.authService.isAuthenticated() };
}
}
// auth.service.ts
import { Injectable, forwardRef, Inject } from '@angular/core';
import { UserService } from './user.service';
@Injectable()
export class AuthService {
constructor(@Inject(forwardRef(() => UserService)) private userService: UserService) {}
isAuthenticated() {
return true;
}
}
3. Refactoring with a Mediator Service
Introduce a third service to mediate between the two dependent services or components.
// mediator.service.ts
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class MediatorService {
isAuthenticated = false;
userData = { name: '', email: '' };
setAuthStatus(status: boolean): void {
this.isAuthenticated = status;
}
setUserData(data: any): void {
this.userData = data;
}
}
// user.service.ts
import { Injectable } from '@angular/core';
import { MediatorService } from './mediator.service';
@Injectable()
export class UserService {
constructor(private mediator: MediatorService) {}
getUserDetails() {
if (this.mediator.isAuthenticated) {
return this.mediator.userData;
}
return null;
}
updateUserData(data: any) {
this.mediator.setUserData(data);
}
}
// auth.service.ts
import { Injectable } from '@angular/core';
import { MediatorService } from './mediator.service';
@Injectable()
export class AuthService {
constructor(private mediator: MediatorService) {}
login(credentials: any): boolean {
// Authentication logic
const isAuthenticated = true; // simplified
this.mediator.setAuthStatus(isAuthenticated);
return isAuthenticated;
}
isAuthenticated(): boolean {
return this.mediator.isAuthenticated;
}
}
4. Event-Based Communication
Use Angular's EventEmitter or RxJS Subjects to implement a pub/sub pattern.
// event.service.ts
import { Injectable } from '@angular/core';
import { Subject } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class EventService {
private authStatusChanged = new Subject<boolean>();
private userDataChanged = new Subject<any>();
authStatus$ = this.authStatusChanged.asObservable();
userData$ = this.userDataChanged.asObservable();
emitAuthStatus(status: boolean): void {
this.authStatusChanged.next(status);
}
emitUserData(data: any): void {
this.userDataChanged.next(data);
}
}
// user.service.ts
import { Injectable } from '@angular/core';
import { EventService } from './event.service';
@Injectable()
export class UserService {
private userData: any = null;
constructor(private eventService: EventService) {
this.eventService.authStatus$.subscribe(status => {
if (!status) {
this.userData = null;
}
});
}
updateUserProfile(data: any) {
this.userData = data;
this.eventService.emitUserData(data);
}
}
// auth.service.ts
import { Injectable } from '@angular/core';
import { EventService } from './event.service';
@Injectable()
export class AuthService {
private isAuthenticated = false;
constructor(private eventService: EventService) {
this.eventService.userData$.subscribe(data => {
console.log('User data updated:', data);
});
}
login(credentials: any): boolean {
// Authentication logic
this.isAuthenticated = true; // simplified
this.eventService.emitAuthStatus(this.isAuthenticated);
return this.isAuthenticated;
}
logout(): void {
this.isAuthenticated = false;
this.eventService.emitAuthStatus(this.isAuthenticated);
}
}
5. State Management Solutions
Using NgRx or Angular's Signals can help eliminate the need for circular references by centralizing state management.
Using Angular Signals (Angular 16+):
// auth.state.ts
import { Injectable, signal } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class AuthState {
isAuthenticated = signal(false);
currentUser = signal<any>(null);
setAuthStatus(status: boolean) {
this.isAuthenticated.set(status);
}
setCurrentUser(user: any) {
this.currentUser.set(user);
}
}
// user.service.ts
import { Injectable } from '@angular/core';
import { AuthState } from './auth.state';
@Injectable()
export class UserService {
constructor(private authState: AuthState) {}
getUserProfile() {
if (this.authState.isAuthenticated()) {
return this.authState.currentUser();
}
return null;
}
updateUserProfile(userData: any) {
this.authState.setCurrentUser(userData);
}
}
// auth.service.ts
import { Injectable } from '@angular/core';
import { AuthState } from './auth.state';
@Injectable()
export class AuthService {
constructor(private authState: AuthState) {}
login(credentials: any): boolean {
// Authentication logic
const isAuthenticated = true; // simplified
const userData = { id: 1, name: 'John Doe' };
this.authState.setAuthStatus(isAuthenticated);
this.authState.setCurrentUser(userData);
return isAuthenticated;
}
logout(): void {
this.authState.setAuthStatus(false);
this.authState.setCurrentUser(null);
}
}
Real-World Example: Dashboard with User Authentication
Let's look at a real-world example where a dashboard needs to show user-specific data, but the authentication logic is separate.
Problem: Cyclic Dependency
// dashboard.component.ts
import { Component } from '@angular/core';
import { UserService } from '../services/user.service';
@Component({
selector: 'app-dashboard',
template: `
<div *ngIf="isAuthenticated">
<h1>Welcome, {{ userData?.name }}</h1>
<div class="dashboard-content">
<!-- Dashboard content here -->
</div>
</div>
<div *ngIf="!isAuthenticated">Please login to view dashboard</div>
`
})
export class DashboardComponent {
isAuthenticated = false;
userData: any = null;
constructor(private userService: UserService) {
this.isAuthenticated = this.userService.isAuthenticated();
this.userData = this.userService.getUserData();
}
}
// user.service.ts
import { Injectable } from '@angular/core';
import { AuthService } from './auth.service';
@Injectable()
export class UserService {
constructor(private authService: AuthService) {}
isAuthenticated(): boolean {
return this.authService.isAuthenticated();
}
getUserData() {
if (this.isAuthenticated()) {
return { name: 'John Doe', role: 'Admin' };
}
return null;
}
}
// auth.service.ts
import { Injectable } from '@angular/core';
import { DashboardComponent } from '../components/dashboard.component';
@Injectable()
export class AuthService {
private authenticated = false;
constructor(private dashboard: DashboardComponent) {} // Cyclic dependency!
isAuthenticated(): boolean {
return this.authenticated;
}
login(credentials: any): boolean {
// Authentication logic
this.authenticated = true;
this.dashboard.isAuthenticated = true; // Updating the component directly
return true;
}
}
Solution: Using State Management and Proper Component Communication
// auth.state.ts
import { Injectable } from '@angular/core';
import { BehaviorSubject } from 'rxjs';
export interface UserData {
name: string;
role: string;
}
@Injectable({
providedIn: 'root'
})
export class AuthState {
private authStatus = new BehaviorSubject<boolean>(false);
private userData = new BehaviorSubject<UserData | null>(null);
authStatus$ = this.authStatus.asObservable();
userData$ = this.userData.asObservable();
setAuthStatus(status: boolean): void {
this.authStatus.next(status);
}
setUserData(data: UserData | null): void {
this.userData.next(data);
}
getCurrentAuthStatus(): boolean {
return this.authStatus.value;
}
getCurrentUserData(): UserData | null {
return this.userData.value;
}
}
// dashboard.component.ts
import { Component, OnInit, OnDestroy } from '@angular/core';
import { AuthState, UserData } from '../services/auth.state';
import { Subscription } from 'rxjs';
@Component({
selector: 'app-dashboard',
template: `
<div *ngIf="isAuthenticated">
<h1>Welcome, {{ userData?.name }}</h1>
<div class="dashboard-content">
<!-- Dashboard content here -->
</div>
</div>
<div *ngIf="!isAuthenticated">Please login to view dashboard</div>
`
})
export class DashboardComponent implements OnInit, OnDestroy {
isAuthenticated = false;
userData: UserData | null = null;
private subscriptions: Subscription[] = [];
constructor(private authState: AuthState) {}
ngOnInit(): void {
// Subscribe to auth status changes
this.subscriptions.push(
this.authState.authStatus$.subscribe(status => {
this.isAuthenticated = status;
})
);
// Subscribe to user data changes
this.subscriptions.push(
this.authState.userData$.subscribe(data => {
this.userData = data;
})
);
// Initialize with current values
this.isAuthenticated = this.authState.getCurrentAuthStatus();
this.userData = this.authState.getCurrentUserData();
}
ngOnDestroy(): void {
// Clean up subscriptions
this.subscriptions.forEach(sub => sub.unsubscribe());
}
}
// user.service.ts
import { Injectable } from '@angular/core';
import { AuthState, UserData } from './auth.state';
@Injectable({
providedIn: 'root'
})
export class UserService {
constructor(private authState: AuthState) {}
getUserData(): UserData | null {
return this.authState.getCurrentUserData();
}
updateUserData(data: UserData): void {
this.authState.setUserData(data);
}
}
// auth.service.ts
import { Injectable } from '@angular/core';
import { AuthState } from './auth.state';
@Injectable({
providedIn: 'root'
})
export class AuthService {
constructor(private authState: AuthState) {}
isAuthenticated(): boolean {
return this.authState.getCurrentAuthStatus();
}
login(credentials: any): boolean {
// Authentication logic
const isAuthenticated = true; // simplified
if (isAuthenticated) {
this.authState.setAuthStatus(true);
this.authState.setUserData({
name: 'John Doe',
role: 'Admin'
});
}
return isAuthenticated;
}
logout(): void {
this.authState.setAuthStatus(false);
this.authState.setUserData(null);
}
}
Best Practices for Preventing Cyclic Dependencies
- Follow Unidirectional Data Flow - Design your application to have a clear direction of data flow, usually from parent to child components.
- Single Responsibility Principle - Ensure each service or component has a single, well-defined responsibility.
- Hierarchical Dependency Structure - Organize your code with a clear hierarchy where high-level modules depend on low-level modules, not the other way around.
- Utilize State Management - For complex applications, use state management patterns like NgRx, Signals, or simpler BehaviorSubject-based solutions.
- Abstract Common Dependencies - Create abstraction layers when multiple components need to interact.
- Code Reviews and Architecture Planning - Regularly review your dependency graph and plan your architecture before implementation.
- Use Dependency Visualization Tools - Tools like Webpack Analyzer can help visualize your dependency structure.
Conclusion
Cyclic dependencies in Angular can lead to complex, hard-to-debug issues, but they're also solvable with the right approaches. By understanding the causes and implementing structured solutions like interface segregation, forwardRef(), mediator services, event-based communication, or state management, you can maintain a clean and maintainable Angular codebase.
When encountering circular dependency errors, take the time to refactor your code properly rather than using quick fixes that might lead to technical debt. With careful architecture planning and adherence to best practices, you can prevent most cyclic dependencies before they occur.
Remember that the best solution depends on your specific use case – sometimes a simple forwardRef() is sufficient, while complex applications might benefit from a comprehensive state management approach.